Python は、最近最も広く使われている汎用プログラミング言語の 1 つです。 ファイル関連のタスクを実行するための多くの組み込みモジュール、関数、キーワードを提供しています。 グロブとは、UNIXのシェル関連のルールに従って特定のパターンにマッチさせるための技術を指す言葉です。 LinuxやUNIXベースのオペレーティングシステムでは、与えられたパターンに従ってファイルやディレクトリを検索するためのglob()関数が提供されています。 Pythonにも、指定されたパターンに一致するファイルやパス名にアクセスしたり、取得したりするための組み込みのglobモジュールが用意されています。 この記事で説明する作業では、Pythonのglobモジュールのglob()関数を使用します。 この記事では、glob()関数を使用して、指定されたパターンに従ってパス名やファイル名を検索する方法を説明します。
例1:ファイル名またはパス名を絶対パスでマッチする
glob()関数の機能と動作を理解するためにいくつかの例を見てみましょう。 まず、ファイル名またはパス名と絶対パスを一致させる簡単な例から始めます。 ファイル名やパス名が絶対パスにマッチする場合、glob()関数はリストの形でマッチを返し、そうでない場合は空のリストを返します。
import glob
#glob関数を使ってパス名と絶対パスをマッチング
#matching absolute path of downloads directory
print(glob.glob(“/home/linuxhint/Downloads”))
#matching absolute path of documents directory
print(glob./home/linuxhint/Downloads))
#import glob(/home/linuxhint/Downloads))
#import glob(1046)を用いてパス名を絶対パスでマッチング。glob(“/home/linuxhint/Documents”))
#Matching absolute path of Desktop
print(glob.glob(“/home/linuxhint/Desktop”))
#matching absolute path of files
print(glob.glob(“/home/linuxhint/Desktop/script.sh”))
print(glob.glob./home/linuxhint/Desktop/script.sh”))
#matching absolutely path of files
print(glob.glob(“/home/linuxhint/Desktop”)))
#matching absolutely path of devicesprint(glob.glob(“/home/linuxhint/Documents/calculator.py”))
#specifying path of file that exists
#The glob function will return the empty list
print(glob.glob(“/home/linuxhint/Documents/myfile.py”))
出力
出力はマッチを示します。
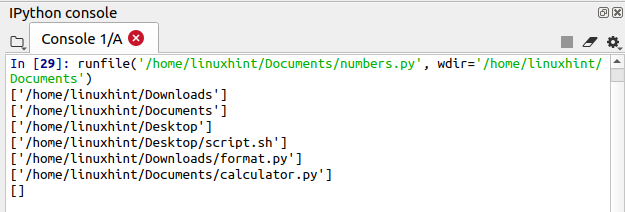
例 2: パス検索にワイルドカードを使用
パス検索のために glob() 関数とワイルド カードを使用できることがあります。 最も一般的に使用されるワイルドカードは、アスタリスク (*)、クエスチョンマーク (?)、数値範囲、およびアルファベット範囲です。
Using an Asterisk (*) Wildcard for Path Retrieval
The asterisk wild card operator is used to match zero or more characters of the absolute path.This uses asterisk (*) Wildcard in the glob() function in the first, we discuss the asterisk use of the glob() function.This will use asterisk in the first, we will discuss the first. アスタリスクで指定された文字がない場合、指定されたパスのすべてのファイル、ディレクトリ、サブディレクトリの絶対パスをリストアップする。 また、アスタリスクにいくつかの文字を記述すると、その文字に基づいた絶対パスをマッチングする。 例えば、.txt ファイルの絶対パスを見つける必要がある場合、* ワイルドカードは *.txt.
これを Python スクリプトで実装します。
import glob
#finding the absolute path of files and directories
print(glob.glob(“/home/linuxhint/Downloads/*”))
print(“————————“)
#finding the absolute path of the .txt files in the Desktop directory
print(glob.glob(“/home/linuxhint/Desktop/*.txt”))
print(“————————“)
#finding the absolute path of the .txt files in the desktop directory
print(“————————“)”—————————————-“)
#finding the absolute path of the .txt files in the Desktop directory
print(“—————————————-“)”—————————————-“(————————————————-)Desktopディレクトリの.shファイルの絶対パス検索
print(glob.glob(“/home/linuxhint/Desktop/*.sh”))
print(“—————————-“)
#Documentsディレクトリの.pyファイルの絶対パス検索
print(glob.glob(“/home/linuxhint/Documents/*.sh”)))
#Finished the absolute path of the.py files in the Document directory.py”))
print(“—————————————-“)
出力
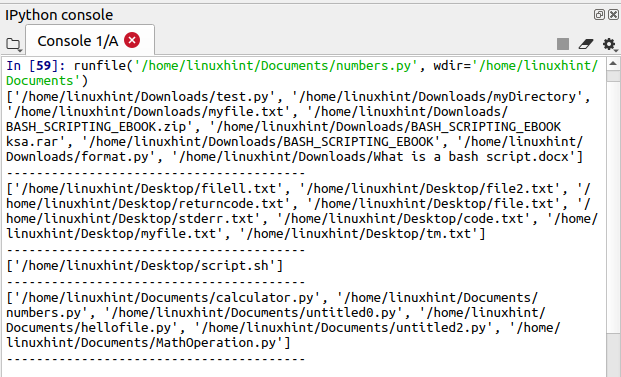
Using a Question Mark (?) Wildcard Operator
疑問符 (?) ワイルドカード演算子は1文字にマッチするように使われるものです。 これは、与えられた名前に含まれる 1 文字を認識できない状況で役立ちます。
これを Python スクリプトで実装します。
import glob
#finding the file with * wildcard operator
print(glob.glob(“/home/linuxhint/Desktop/file?.txt”))
出力
マッチしたファイルを表示します。
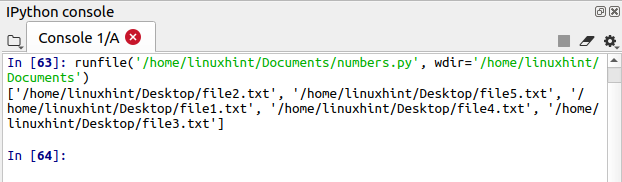
範囲ワイルドカード演算子を使用する
文字または数字の与えられた範囲内のファイルにマッチするには、範囲ワイルドカード演算子を使用します。 範囲は角括弧の中で定義します。
範囲を定義することによって、ファイルの絶対パスを見つけます。
import glob
#find the absolute path of files in a given range
#defining the range in characters
print(glob.glob(“/home/linuxhint/Desktop/*”))
#print(dotted line to differentiate the output
print(“——————————————“)
#defining the range in numbers
print(glob.glob(“/home/linuxhint/Desktop/*”))
出力
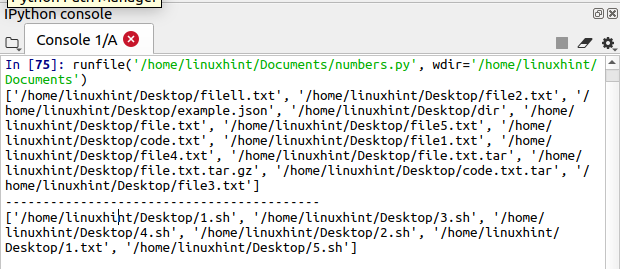
Find Files Recursively Using glob()
glob関数は引数として2つのパラメーターをとります。 最初のパラメータはパス名を定義し、2番目のパラメータは再帰的なプロパティを定義する。 2番目のパラメータはオプションで、recursiveプロパティはデフォルトで “false “に設定されています。 しかし、recursiveプロパティを「true」に設定すると、ファイルパスを再帰的に見つけることができます。
このPythonスクリプトでrecursiveプロパティを「true」に設定し、ファイルの絶対パスを再帰的に見つけることにします。
import glob
#finding the files recursively
print(glob.glob(“/home/linuxhint/Documents/*”,recursive=True))
Output
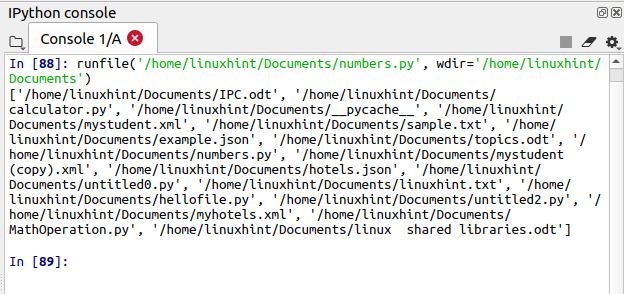
結論
Glob とは、UNIX シェル関連の規則に従って特定のパターンにマッチするために使われる技術を指す共通用語である。 Pythonは、与えられたルールに従ってパス名にアクセスするための組み込みのglobモジュールと関数を提供します。 この記事では、glob()関数を使ってパス名を見つける方法を、さまざまな例を使って説明します。